The Complete Guide to Building a JavaScript Slot Machine: From Concept to Finished Game
- AIS Technolabs
- Apr 1
- 5 min read
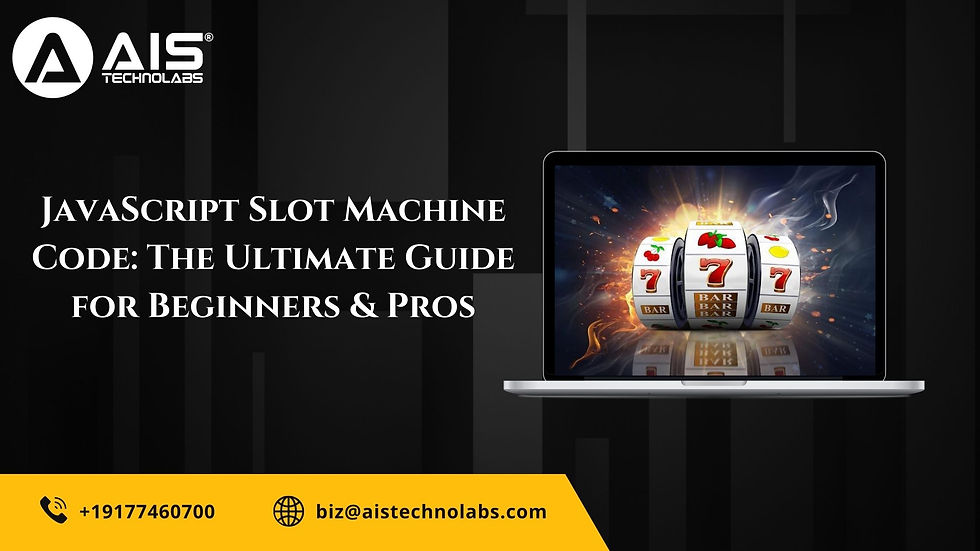
Introduction to JavaScript Slot Machine Development
Slot machines have been a casino staple for over a century, and with JavaScript Slot Machine Code, you can recreate this classic gaming experience for the web. This comprehensive guide will walk you through every aspect of developing a fully functional slot machine using JavaScript Slot Machine Code, covering both fundamental concepts and advanced techniques. Building a slot machine in JavaScript provides an excellent opportunity to master several key web development skills:
Working with random number generation
Implementing smooth animations and transitions
Creating interactive UI elements
Developing game logic and win conditions
Ensuring responsive design across devices
Whether you're a beginner looking to expand your JavaScript knowledge or an experienced developer wanting to create casino-style games, this guide will provide the complete roadmap.
Understanding Slot Machine Mechanics
Core Components of a Slot Machine
Every slot machine consists of three primary elements:
Visual Display Elements
Reels that spin and display symbols
Paylines that determine winning combinations
Information displays for credits, wins, and bets
Game Logic Components
Random number generation for symbol selection
Win condition evaluation
Payout calculations
User Interface Controls
Spin button
Bet adjustment controls
Game settings and information
How Slot Machines Work Mathematically
Modern slot machines operate on the principle of random number generation (RNG). When a player presses the spin button:
The RNG selects random positions for each reel
The reels animate to these positions
The game evaluates the final symbol combination
Winnings are calculated based on pay tables
The key to an engaging slot machine is balancing:
Frequency of wins (hit rate)
Size of payouts
Overall return to player percentage
Planning Your JavaScript Slot Machine
Determining Game Specifications
Before writing any code, you should define:
Reel and Symbol Configuration
Number of reels (typically 3-5)
Number of symbols per reel
Symbol types and their relative frequencies
Paytable Structure
Winning symbol combinations
Payout multipliers for each combination
Special symbols (wilds, scatters, bonuses)
Game Features
Basic spins
Bonus rounds
Free spins
Progressive jackpots
User Experience Considerations
A well-designed slot machine should:
Provide clear visual feedback during spins
Display win information prominently
Include intuitive controls
Maintain consistent performance across devices
Offer appropriate sound effects and animations
Developing the Visual Interface
Designing the Reel Display
The reel display is the centerpiece of your slot machine. Key design elements include:
Reel Container
Fixed width and height
Distinctive border styling
Proper spacing between reels
Symbol Design
Visually distinct symbols
Consistent sizing
Clear hierarchy (higher-value symbols should stand out)
Animation Effects
Smooth spinning motion
Realistic deceleration
Winning symbol highlights
Creating the Control Panel
The control panel typically contains:
Spin Button
Prominent placement
Clear visual states (normal, pressed, disabled)
Responsive feedback when clicked
Bet Controls
Buttons to increase/decrease bet
Current bet display
Bet limits indication
Game Information
Credit balance
Last win amount
Paytable access
Implementing Game Logic
Random Number Generation
The foundation of slot machine fairness is proper random number generation. JavaScript provides several approaches:
Basic Randomization
Using Math.random() for simple implementations
Limitations in true randomness for serious applications
Advanced RNG Techniques
Cryptographic randomization for better unpredictability
Seed-based random number generation
Server-side RNG for commercial applications
Reel Spin Mechanics
Implementing realistic reel spinning involves:
Spin Initiation
Starting all reels simultaneously
Applying initial spin acceleration
Spin Animation
Smooth movement through symbol positions
Variable speed for realistic effect
Synchronized reel stopping
Final Position Determination
Mapping RNG results to symbol positions
Ensuring valid stopping points
Handling symbol weighting
Win Detection and Payouts
The win evaluation system must:
Check All Active Paylines
Horizontal lines
Diagonal lines
Zig-zag patterns
Scatter pays
Calculate Payouts
Apply payable multipliers
Handle special symbol effects
Accumulate multiple wins
Update Player Balance
Add winnings to credit total
Deduct bets when appropriate
Handle bonus round entries
Advanced Features and Enhancements
Bonus Rounds and Special Features
To increase player engagement, consider adding:
Free Spin Modes
Triggered by specific symbol combinations
Multiplied winnings during free spins
Retrigger possibilities
Pick-and-Win Bonuses
Interactive mini-games
Instant prize awards
Progressive bonus values
Wild Features
Expanding wilds
Sticky wilds
Shifting wilds
Progressive Jackpots
For more sophisticated implementations:
Standalone Progressives
Grows with bets on single machine
Fixed reset amount
Linked Progressives
Multiple machines contribute
Networked jackpot growth
Larger potential payouts
Performance Optimization Techniques
Animation Efficiency
Ensure smooth performance by:
Using CSS transforms instead of position changes
Implementing request Animation Frame for JavaScript animations
Optimizing sprite sheets for symbols
Reducing repaints and reflows during spins
Memory Management
JavaScript games can suffer from memory leaks if not properly managed:
Clearing unused event listeners
Properly disposing of animation objects
Managing sound effect resources
Implementing object pooling where appropriate
Testing and Debugging Strategies
Comprehensive Test Cases
Develop a testing plan covering:
Basic Functionality
Spin initiation and completion
Win detection accuracy
Payout calculations
Edge Cases
Minimum and maximum bets
Consecutive wins
Empty credit scenarios
Performance Testing
Load testing with rapid spins
Memory usage over extended play
Cross-browser compatibility
Debugging Techniques
Effective debugging approaches include:
Console logging of game state changes
Visual debugging of reel positions
Unit testing for core algorithms
Player simulation for stress testing
Legal and Ethical Considerations
Gambling Regulations
Important legal aspects to consider:
Jurisdictional Laws
Varying regulations by country/state
Age restrictions
Prize limitations
Fairness Requirements
RNG certification needs
Payout percentage disclosures
Game auditing standards
Responsible Gaming Features
For ethical implementations:
Player Protection Tools
Session time limits
Loss limits
Self-exclusion options
Transparent Information
Clear odds displays
Paytable accessibility
Game rules documentation
Monetization Strategies
Virtual Currency Models
Common approaches include:
Demo Mode with Ad Support
Free-to-play with advertisements
Optional ad removal purchases
In-App Purchases
Virtual coin packages
Special feature unlocks
Cosmetic upgrades
Real Money Considerations
For regulated markets:
Payment Processing
Secure transaction handling
Multiple payment methods
Fraud prevention
Player Verification
Age confirmation
Identity checks
Location validation
Conclusion: Bringing It All Together
Building a complete JavaScript slot machine involves combining multiple technical disciplines into a cohesive gaming experience. From the visual design of spinning reels to the mathematical models determining payout frequencies, each component plays a crucial role in creating an engaging and fair game.
For developers looking to create commercial-grade slot machine games, AIS Technolabs offers professional game development services with expertise in casino-style gaming implementations. Contact us for customized gaming solutions tailored to your specific requirements.
Frequently Asked Questions
1. How difficult is it to build a slot machine with JavaScript?
The basic functionality can be implemented by intermediate JavaScript developers, but advanced features require more sophisticated programming skills.
2. Can I create a slot machine without using canvas?
Yes, modern CSS animations combined with DOM manipulation can create excellent slot machine effects without canvas.
3. What's the most challenging part of slot machine development?
Implementing realistic physics for reel spinning while maintaining precise control over symbol positioning often proves most challenging.
4. How do I ensure my slot machine is fair?
Use properly implemented random number generation and consider third-party auditing for commercial applications.
5. Can I add multiplayer features to a JavaScript slot machine?
While possible, multiplayer functionality requires server-side integration and significantly increases complexity.
6. How important are sound effects in slot machine design?
Audio feedback is crucial for player engagement, providing important cues about wins and game events.
7. What browsers should I test my slot machine on?
Prioritize Chrome, Firefox, Safari, and Edge, with particular attention to mobile browser performance.
8. How can I make my slot machine stand out?
Unique themes, innovative bonus features, and polished animations help differentiate your implementation.
View source : https://aistechnolabs.weebly.com/blog1/the-complete-guide-to-building-a-javascript-slot-machine-from-concept-to-finished-game
תגובות